Determining body mass index (BMI) is crucial for health assessments. JavaScript offers efficient methods for calculating BMI, especially with the advent of modern web applications.
BMI, a metric assessing body fat based on height and weight, has gained widespread relevance in healthcare. It classifies individuals into weight categories, aiding in understanding obesity risk and associated health concerns. Historically, BMI originated as the Quetelet Index, developed by Adolphe Quetelet in the 1830s.
This article will delve into the intricacies of calculating BMI using JavaScript, exploring various approaches and their applications in real-world scenarios.
How to Calculate BMI using JavaScript
Understanding the key aspects of calculating BMI using JavaScript is essential for developing accurate and reliable applications.
- Formula: BMI = weight (kg) / height (m2)
- Units: Weight in kilograms, height in meters
- Categories: Underweight, normal, overweight, obese
- Health Implications: Obesity risk assessment
- JavaScript Functions: Math.pow(), Math.round()
- Input Validation: Checking for valid weight and height values
- Error Handling: Managing invalid inputs or calculation errors
- User Interface: Displaying BMI results and category
These aspects are interconnected, forming the foundation for effective BMI calculation. For instance, the formula dictates the mathematical operation, while JavaScript functions facilitate the calculations. Input validation ensures reliable results, and user interface elements provide a seamless user experience. Together, these aspects enable the development of robust and informative BMI calculators using JavaScript.
Formula
The formula BMI = weight (kg) / height (m2) lies at the core of BMI calculation using JavaScript. It establishes the mathematical relationship between weight and height, enabling the quantification of body mass index.
- Weight (kg)
Weight, measured in kilograms (kg), represents the gravitational force acting on an individual’s mass. Accurate weight measurement is crucial for precise BMI calculations.
- Height (m2)
Height, expressed in meters squared (m2), signifies the area occupied by an individual. Calculating height accurately involves converting height measurements to meters and then squaring the value to obtain the area.
- Units of Measurement
Utilizing consistent units of measurement is essential. Weight should be entered in kilograms (kg), and height should be converted to meters (m) before squaring. Mixing units, such as pounds for weight and feet for height, can lead to incorrect BMI calculations.
- Rounding and Precision
BMI calculations may involve decimal values. For practical purposes, rounding the BMI to one decimal place is often sufficient. However, maintaining precision throughout the calculation process is crucial to ensure accurate results.
Understanding these facets of the BMI formula empowers developers to create JavaScript applications that accurately assess body mass index, providing valuable insights for health and fitness assessments.
Units
In the context of “how to calculate BMI using JavaScript”, the specified units of measurement, weight in kilograms and height in meters, play a critical role in ensuring accurate and reliable results. The BMI formula, BMI = weight (kg) / height (m2), explicitly requires these units for correct calculation.
Using the correct units is essential because the formula is calibrated to produce meaningful BMI values when weight is expressed in kilograms and height is expressed in meters squared. Abweiching from these units can lead to incorrect BMI calculations and misinterpretation of results.
Real-life examples further illustrate the importance of using the correct units. For instance, if weight is entered in pounds and height is entered in feet, the calculated BMI will be incorrect. This is because the formula expects weight in kilograms and height in meters squared, and converting the values later can introduce errors.
Understanding the relationship between units and BMI calculation empowers developers to create JavaScript applications that accurately assess body mass index, providing valuable insights for health and fitness assessments. Adhering to the specified units ensures that the calculated BMI is meaningful and reliable for making informed decisions.
Categories
Categorizing individuals based on BMI is a crucial aspect of interpreting BMI calculations. These categories provide valuable insights into health risks associated with different weight ranges.
- Underweight
Individuals with BMI below 18.5 are considered underweight. This category may indicate malnutrition or underlying health conditions and can be associated with increased risk of certain diseases.
- Normal
Individuals with BMI between 18.5 and 24.9 are considered to have a healthy weight. This range is generally associated with lower risk of chronic diseases and optimal overall health.
- Overweight
Individuals with BMI between 25 and 29.9 are considered overweight. This category may indicate excess body fat and can increase the risk of developing weight-related health conditions.
- Obese
Individuals with BMI of 30 or higher are considered obese. Obesity is a major risk factor for various chronic diseases, including heart disease, stroke, type 2 diabetes, and some types of cancer.
Understanding these categories helps individuals assess their weight status and make informed decisions about lifestyle changes to maintain a healthy weight. By incorporating BMI categories into JavaScript applications, developers can provide users with personalized insights and empower them to take proactive steps towards improving their health and well-being.
Health Implications
BMI plays a pivotal role in assessing obesity risk, a major public health concern. JavaScript-based BMI calculators can provide valuable insights into potential health implications related to weight status.
- Chronic Disease Risk
Obesity increases the likelihood of developing chronic diseases such as heart disease, stroke, type 2 diabetes, and certain types of cancer. BMI serves as an indicator of this risk, allowing individuals to take proactive measures.
- Cardiovascular Health
Excess weight strains the cardiovascular system, increasing the risk of heart disease and stroke. BMI helps identify individuals at risk, enabling early intervention and lifestyle modifications to improve heart health.
- Metabolic Syndrome
Obesity is a key component of metabolic syndrome, a cluster of conditions that increase the risk of heart disease, stroke, and type 2 diabetes. BMI can help identify individuals with metabolic syndrome, prompting further evaluation and management.
- Quality of Life
Obesity can impair physical mobility, reduce energy levels, and affect mental well-being. BMI can indicate potential health concerns that may impact quality of life, encouraging individuals to seek support and make positive lifestyle changes.
Understanding the health implications associated with obesity risk assessment empowers individuals to make informed choices about their weight management. JavaScript-based BMI calculators provide a readily accessible tool for assessing obesity risk, promoting preventive healthcare and encouraging healthier lifestyles.
JavaScript Functions
In the context of “how to calculate BMI using JavaScript,” the functions Math.pow() and Math.round() play critical roles in performing precise and accurate calculations.
- Calculating Height Squared
Math.pow() is utilized to square the height value, which is expressed in meters. This operation is essential for calculating BMI as per the formula: BMI = weight (kg) / height (m2).
- Rounding BMI Value
Math.round() is employed to round the calculated BMI value to one decimal place. This step ensures consistency and readability of the BMI results.
- Error Handling
JavaScript functions provide mechanisms for error handling, such as checking for invalid inputs or calculation errors. This ensures the robustness and reliability of the BMI calculator.
- Cross-Platform Compatibility
JavaScript functions like Math.pow() and Math.round() are widely supported across different platforms and browsers, ensuring the consistent calculation of BMI regardless of the environment.
Understanding these functions and their applications empowers developers to create JavaScript-based BMI calculators that deliver accurate and reliable results. These calculators can be embedded in various health and fitness applications, enabling users to conveniently assess their BMI and make informed decisions about their weight management.
Input Validation
Input validation is a critical aspect of “how to calculate BMI using JavaScript” as it ensures the accuracy and reliability of the results. It involves checking for valid weight and height values to prevent errors and produce meaningful BMI calculations.
- Value Range Validation
This involves checking if the entered weight and height values fall within expected ranges. For example, negative weight values or extreme height values should be flagged as invalid.
- Data Type Verification
It is essential to ensure that the entered values are of the correct data type. For instance, weight should be a numeric value representing kilograms, and height should be a numeric value representing meters.
- Missing or Empty Values
Input validation should handle cases where users leave weight or height fields empty. Such missing values can lead to calculation errors and should be addressed accordingly.
- Error Handling and Feedback
When invalid values are encountered, the calculator should provide clear error messages or feedback to the user. This helps users identify and correct errors, ensuring accurate BMI calculations.
Input validation plays a vital role in developing robust and reliable JavaScript-based BMI calculators. By implementing these checks, developers can prevent erroneous results, improve user experience, and ensure the accuracy of BMI calculations for health and fitness assessments.
Error Handling
In the context of “how to calculate BMI using JavaScript,” error handling is a crucial aspect that ensures the accuracy and reliability of BMI calculations. It involves managing invalid inputs or calculation errors that may arise during the process.
Error handling plays a critical role as a component of “how to calculate BMI using JavaScript” because it prevents incorrect or misleading BMI results. Invalid inputs, such as negative weight values or non-numeric height values, can lead to erroneous calculations. Additionally, calculation errors, such as division by zero when height is zero, must be handled to avoid unexpected behavior.
Real-life examples of error handling in “how to calculate BMI using JavaScript” include:
- Checking for empty or missing weight or height values before performing calculations.
- Validating that entered values are within expected ranges to prevent unrealistic results.
- Handling calculation errors by displaying informative error messages to the user.
Understanding the practical applications of error handling in “how to calculate BMI using JavaScript” empowers developers to create robust and user-friendly BMI calculators. By implementing effective error handling mechanisms, developers can prevent incorrect results, improve user experience, and ensure the accuracy of BMI calculations for health and fitness assessments.
User Interface
In the context of “how to calculate BMI using JavaScript,” the user interface (UI) plays a pivotal role in presenting the calculated BMI results and categorizing them for better understanding. The UI serves as a crucial component of the BMI calculator, as it enables users to interact with the application, view the results, and interpret their significance.
A well-designed UI should effectively display the calculated BMI value, typically rounded to one decimal place, along with a corresponding BMI category (e.g., underweight, normal, overweight, obese). This categorization helps users quickly understand their weight status and potential health implications. Additionally, the UI should provide clear instructions on how to use the calculator and interpret the results.
Real-life examples of “User Interface: Displaying BMI results and category” within “how to calculate BMI using JavaScript” include:
- Interactive BMI calculators on health and fitness websites that allow users to input their weight and height and instantly receive their BMI and category.
- Mobile applications that incorporate BMI calculators, enabling users to track their weight status over time and set goals for weight management.
- Healthcare professionals using BMI calculators during patient consultations to assess weight status and provide personalized recommendations.
Understanding the practical applications of the UI in “how to calculate BMI using JavaScript” empowers developers to create user-friendly and informative BMI calculators. By designing an effective UI, developers can enhance the user experience, promote accurate interpretation of BMI results, and contribute to improved health outcomes.
Frequently Asked Questions on How to Calculate BMI using JavaScript
This section addresses commonly asked questions or clarifies aspects of BMI calculation using JavaScript.
Question 1: What is JavaScript’s role in calculating BMI?
JavaScript provides functions to perform mathematical calculations, including BMI calculations, using the formula BMI = weight(kg) / height(m2).
Question 2: How can I ensure accurate BMI calculations?
To ensure accuracy, use correct units (kg for weight, m for height), validate input data for validity and range, and handle calculation errors.
Question 3: How do I display the BMI result and category?
Use a user-friendly interface to display the calculated BMI, rounded to one decimal place, along with the corresponding BMI category (e.g., underweight, normal, overweight, obese).
Question 4: Can I use JavaScript to calculate BMI in different units?
Yes, but ensure proper unit conversion. Convert weight to kilograms if it’s in pounds, and convert height to meters if it’s in feet or inches.
Question 5: How can I handle invalid inputs or errors?
Implement error handling to check for empty inputs, invalid values, or calculation errors. Display clear error messages to guide users in correcting the issue.
Question 6: What are the limitations of using JavaScript for BMI calculations?
JavaScript’s BMI calculations are limited by the accuracy of the input data and the potential for rounding errors. It’s essential to note that BMI is an indicator of weight status and should be interpreted in conjunction with other health assessments.
These FAQs provide insights into the practical aspects of calculating BMI using JavaScript. Understanding these concepts is crucial for developing accurate and user-friendly BMI calculators.
Now, let’s delve into the implementation details of BMI calculation in JavaScript, exploring the code structure and best practices.
Essential Tips for Calculating BMI using JavaScript
To ensure accurate and reliable BMI calculations, consider these essential tips:
Tip 1: Validate Input Data
Check for empty or invalid weight and height values before performing calculations.
Tip 2: Use Correct Units
Ensure that weight is in kilograms and height is in meters for precise BMI calculations.
Tip 3: Handle Calculation Errors
Implement error handling to gracefully manage division by zero or other calculation errors.
Tip 4: Round BMI Value
Round the calculated BMI value to one decimal place for consistency and readability.
Tip 5: Display BMI Category
Classify the BMI into appropriate categories (e.g., underweight, normal, overweight, obese) to aid interpretation.
Tip 6: Provide User Feedback
Use clear error messages or feedback to inform users about invalid inputs or calculation issues.
Summary: By implementing these tips, developers can create robust and user-friendly BMI calculators in JavaScript, ensuring accurate BMI calculations for health and fitness assessments.
These tips lay the foundation for the concluding section, which will delve into advanced techniques and considerations for developing comprehensive BMI calculators using JavaScript.
Conclusion
This article has provided a comprehensive exploration of “how to calculate BMI JavaScript,” delving into the intricacies of BMI calculation, its significance, and practical implementation using JavaScript. Key insights include understanding the BMI formula, utilizing JavaScript functions for accurate calculations, and incorporating input validation and error handling for robust applications.
The central points to consider are: (1) The formula, units, and categories used in BMI calculation provide a standardized approach for assessing weight status. (2) JavaScript offers powerful functions for performing these calculations efficiently. (3) Implementing input validation and error handling ensures reliable and user-friendly BMI calculators. These aspects are interconnected and essential for creating effective BMI assessment tools.
In conclusion, understanding “how to calculate BMI JavaScript” empowers developers to create valuable applications for health and fitness assessments. By incorporating the insights and techniques discussed in this article, developers can contribute to the development of accurate, reliable, and user-friendly BMI calculators, promoting healthier lifestyles and improved well-being.
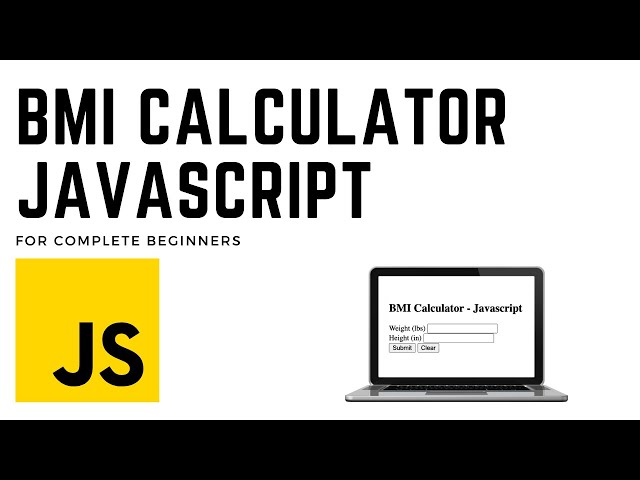