Discount percentage calculation is a core aspect of any e-commerce or financial application. It allows businesses to offer discounts to customers, thereby increasing sales and customer satisfaction. For developers using the ASP.NET C# platform, calculating discount percentages accurately is essential for implementing effective pricing strategies.
The knowledge of discount percentage calculation has been around for centuries. From ancient merchants determining the appropriate reduction in prices to attract buyers to modern-day retailers using sophisticated algorithms, the concept of discounts has shaped the way goods and services are exchanged.
In this article, we will delve into the specifics of calculating discount percentages in ASP.NET C#, providing a step-by-step guide, practical examples, and best practices to ensure accurate and efficient discount calculations in your web applications.
How to Calculate Discount Percentage in ASP.NET C#
Calculating discount percentages accurately in ASP.NET C# is essential for e-commerce and financial applications. Understanding the key aspects involved in this process is crucial for effective implementation.
- Discount Formula
- Percentage Calculation
- ASP.NET C# Syntax
- Data Types
- Rounding
- Error Handling
- Performance Optimization
- Unit Testing
These aspects encompass the core elements of discount percentage calculation in ASP.NET C#, from understanding the mathematical formula to implementing it efficiently in code. By considering these aspects, developers can ensure accurate and reliable discount calculations in their web applications.
Discount Formula
The discount formula is the foundation for calculating discount percentages accurately in ASP.NET C#. It involves understanding the mathematical relationship between the original price, discount rate, and discounted price. By leveraging this formula, developers can programmatically determine the appropriate discount amount for various scenarios in e-commerce and financial applications.
- Original Price: The original price of the item or service before any discounts are applied.
- Discount Rate: The percentage or fixed amount by which the original price is reduced.
- Discounted Price: The resulting price after applying the discount to the original price.
- Discount Percentage: The percentage by which the original price is reduced, calculated as a ratio of the discount rate to the original price.
Understanding these components and their is crucial for accurate discount calculations. The discount formula empowers developers to programmatically implement sophisticated pricing strategies, promotions, and discounts, enhancing the user experience and driving business outcomes.
Percentage Calculation
Percentage calculation plays a critical role in determining discount percentages in ASP.NET C#. It involves calculating the percentage by which the original price of an item or service is reduced. This calculation is essential for offering accurate discounts to customers, ensuring fair pricing, and driving sales.
To calculate the discount percentage, it is necessary to divide the discount amount by the original price and then multiply the result by 100. This process allows developers to determine the percentage reduction in the price. Understanding the relationship between discount amount, original price, and discount percentage is crucial for accurate calculations.
In real-life scenarios, percentage calculation is applied in various ways within the context of discount calculations in ASP.NET C#. For instance, an e-commerce website may offer a 20% discount on a product with an original price of $100. Using the formula mentioned earlier, the discount percentage can be calculated as follows: Discount Percentage = (Discount Amount / Original Price) 100 = (20 / 100) 100 = 20%. This calculation ensures that the customer receives the appropriate discount based on the established discount rate.
By mastering the techniques of percentage calculation, developers can effectively implement discount features in their ASP.NET C# applications. This understanding empowers them to create dynamic pricing strategies, offer personalized discounts to customers, and enhance the overall user experience. Furthermore, accurate discount calculations contribute to customer satisfaction, increased sales, and improved business outcomes.
ASP.NET C# Syntax
ASP.NET C# syntax provides the foundation for writing code that performs discount percentage calculations in ASP.NET applications. Understanding its key elements is crucial for developers seeking to implement accurate and efficient discount mechanisms.
- Data Types: ASP.NET C# supports various data types, including decimal and double, which are commonly used for representing monetary values and percentages in discount calculations.
- Operators: Arithmetic operators such as +, -, *, and / are used to perform mathematical operations on prices and discount rates, enabling the calculation of discounted prices.
- Control Flow: Conditional statements (if-else) and loops (for, while) are used to control the flow of execution, allowing for conditional application of discounts based on specific criteria.
- Formatting: String formatting techniques are employed to display discount percentages and discounted prices in a user-friendly manner, enhancing the presentation of discount information to end-users.
ASP.NET C#
Data Types
In calculating discount percentages in ASP.NET C#, data types play a crucial role in ensuring accurate and efficient calculations. They determine the representation and manipulation of numeric values, including prices and discounts, and influence the precision and reliability of the results.
- Numeric Data Types: Numeric data types, such as decimal and double, are used to store and operate on monetary values. They provide varying degrees of precision and range, allowing developers to choose the most appropriate type for their specific requirements.
- Data Type Conversions: Data type conversions may be necessary to ensure compatibility between different numeric data types. For example, converting a decimal value to a double or vice versa allows for seamless calculations and avoids potential data loss or precision issues.
- Data Type Overflow: When performing calculations, it’s essential to consider the limits of data types. Exceeding the maximum value or minimum value of a data type can lead to overflow or underflow errors, resulting in incorrect discount calculations.
- Data Type Selection: Choosing the appropriate data type is critical for maintaining accuracy and optimizing performance. Selecting a data type with sufficient precision and range ensures that calculations are performed correctly and avoids unnecessary data conversions.
By carefully considering data types and their implications in ASP.NET C# discount percentage calculations, developers can ensure the accuracy, reliability, and efficiency of their code, leading to robust and user-friendly applications.
Rounding
Rounding is a fundamental mathematical operation that plays a crucial role in “how to calculate discount percentage in asp.net c#”. It involves adjusting a numeric value to the nearest whole number, decimal place, or significant digit. Rounding becomes necessary when dealing with calculations that produce fractional results or when a specific level of precision is required.
In the context of discount percentage calculation, rounding is often used to determine the final discounted price. For example, if a product has an original price of $100 and a discount of 15%, the discounted price before rounding would be $85. However, it’s common practice to round the discounted price to the nearest whole number, resulting in a final discounted price of $85. This rounding ensures that the discounted price is presented as a whole number, making it easier for customers to understand and avoid confusion.
Rounding also has practical applications in various scenarios. For instance, in an e-commerce application, rounding can be applied to shipping costs or tax calculations. By rounding these values to the nearest cent or dollar, the application can simplify the checkout process and provide customers with clear and concise pricing information. Additionally, rounding can be used to optimize storage space in databases or improve the performance of mathematical calculations, as it reduces the number of digits that need to be processed.
Understanding the connection between rounding and “how to calculate discount percentage in asp.net c#” is essential for developers seeking to write accurate and efficient code. By leveraging rounding techniques, they can ensure that discount percentages and discounted prices are calculated and presented in a clear and meaningful way, enhancing the user experience and maintaining the integrity of financial transactions.
Error Handling
Error handling is a critical aspect of “how to calculate discount percentage in asp.net c#”, as it allows developers to manage and respond to potential errors that may occur during the calculation process. Errors can arise due to various reasons, such as invalid input data, incorrect formulas, or system failures. By implementing robust error handling mechanisms, developers can ensure that their applications respond gracefully to these errors, providing a better user experience and preventing unexpected behavior.
One of the key benefits of error handling is that it enables developers to identify and isolate the source of the error, making it easier to resolve and prevent similar errors from occurring in the future. In the context of discount percentage calculation, error handling can help identify issues such as invalid discount rates or incorrect product prices, allowing developers to promptly address these issues and maintain the accuracy of their calculations.
Real-life examples of error handling in “how to calculate discount percentage in asp.net c#” include:
- Validating input data: Checking for invalid characters or values in the input data, such as negative discount rates or non-numeric values, and handling these errors gracefully.
- Handling database errors: Managing errors that may occur while accessing or updating the database, such as connection failures or data integrity issues, and ensuring that the discount calculation process is not affected by these errors.
- Catching exceptions: Using exception handling techniques to catch and handle unexpected errors that may occur during the calculation process, providing a clear error message to the user and preventing the application from crashing.
Understanding the connection between error handling and “how to calculate discount percentage in asp.net c#” is essential for developing robust and reliable applications. By implementing effective error handling strategies, developers can ensure that their applications can handle unexpected situations gracefully, providing a better user experience and maintaining the integrity of their calculations.
Performance Optimization
Performance optimization is a crucial aspect of “how to calculate discount percentage in asp.net c#”, as it directly impacts the efficiency and responsiveness of your application. Neglecting performance optimization can lead to slow calculation times, user frustration, and potential business losses. Optimizing the performance of discount percentage calculations ensures that your application runs smoothly, provides a better user experience, and supports scalability as your business grows.
- Efficient Algorithms: Choosing efficient algorithms for discount percentage calculations is paramount. Consider using fast mathematical operations, avoiding unnecessary loops or branching, and optimizing the number of database queries.
- Data Structures: Selecting appropriate data structures for storing and accessing data can significantly impact performance. Utilizing optimized data structures, such as hash tables or sorted lists, allows for faster lookups and reduced calculation time.
- Caching: Caching frequently used values or results can dramatically improve performance. Store calculated discount percentages in a cache to avoid redundant calculations, especially for frequently discounted items.
- Concurrency: If your application handles multiple concurrent requests, implementing proper concurrency mechanisms is essential. This ensures that discount percentage calculations are performed accurately and efficiently, even under high load.
By focusing on these performance optimization techniques, you can ensure that your “how to calculate discount percentage in asp.net c#” implementation meets the demands of your application and provides a seamless user experience. Moreover, performance optimization contributes to the overall efficiency and scalability of your system, setting the foundation for future growth and success.
Unit Testing
Unit testing plays a critical role in ensuring the accuracy and reliability of “how to calculate discount percentage in asp.net c#”. It involves testing individual units of code, such as functions or methods, to verify their behavior and correctness. By testing each unit in isolation, developers can identify and fix errors early on, preventing them from propagating to the entire application. Furthermore, unit testing serves as a safety net, providing confidence in the correctness of the discount percentage calculations, especially when code changes are introduced.
Real-life examples of unit testing in “how to calculate discount percentage in asp.net c#” include:
- Testing the correctness of the discount formula by comparing the calculated discount percentage with expected results.
- Verifying that the code correctly handles edge cases, such as negative discount rates or invalid input values.
- Ensuring that the discount percentage is rounded appropriately according to business rules.
The practical significance of understanding the connection between unit testing and “how to calculate discount percentage in asp.net c#” lies in its impact on the overall quality and reliability of the application. Unit testing helps prevent errors, reduces the risk of incorrect discount calculations, and improves the maintainability of the codebase. By investing in unit testing, developers can ensure that their discount percentage calculations are accurate, consistent, and meet the requirements of the business.
FAQs on Discount Percentage Calculation in ASP.NET C#
This section addresses frequently asked questions (FAQs) related to discount percentage calculation in ASP.NET C#. These FAQs aim to clarify common concerns or misconceptions, providing additional insights and practical guidance.
Question 1: What is the formula for calculating discount percentage?
Answer: The discount percentage formula is: (Discount Amount / Original Price) x 100. This formula calculates the discount as a percentage of the original price.
Question 2: How do I handle negative discount rates or invalid input values?
Answer: It’s important to validate input data and handle edge cases. Check for negative discount rates and non-numeric values, and provide appropriate error messages or default values.
Question 3: What data types should I use for discount calculations?
Answer: For precise calculations, use numeric data types like decimal or double. Consider the range and precision requirements of your application to choose the appropriate data type.
Question 4: How can I improve the performance of my discount calculation code?
Answer: Optimize your code by using efficient algorithms, appropriate data structures, caching, and concurrency mechanisms to handle multiple requests.
Question 5: Why is unit testing important for discount percentage calculations?
Answer: Unit testing helps ensure the accuracy and reliability of your calculations by testing individual units of code in isolation, identifying and fixing errors early on.
Question 6: How do I handle rounding in discount calculations?
Answer: Rounding is often used to present discounted prices as whole numbers. Determine the appropriate rounding rules based on your business requirements and apply them consistently.
These FAQs provide essential insights into discount percentage calculation in ASP.NET C#. By addressing common questions, we aim to empower developers with the knowledge and techniques to implement accurate and efficient discount calculations in their applications.
In the next section, we will delve deeper into advanced techniques and best practices for discount percentage calculation in ASP.NET C#.
Tips for Discount Percentage Calculation in ASP.NET C#
This section provides practical tips to enhance the accuracy, efficiency, and maintainability of discount percentage calculations in your ASP.NET C# applications.
Tip 1: Leverage Decimal Data Type for Precision: Utilize the decimal data type for calculations involving monetary values to ensure precision and minimize rounding errors.
Tip 2: Handle Edge Cases: Validate input values to handle negative discount rates or invalid prices, providing meaningful error messages or default values.
Tip 3: Optimize Performance with Caching: Cache frequently calculated discount percentages to avoid redundant calculations, especially for commonly discounted items.
Tip 4: Implement Unit Testing: Write unit tests to verify the correctness of your discount calculation logic, ensuring accuracy and reliability.
Tip 5: Consider Rounding Rules: Determine appropriate rounding rules based on business requirements and apply them consistently to present discounted prices clearly.
Tip 6: Utilize Efficient Algorithms: Choose efficient mathematical algorithms for discount calculations to minimize processing time and improve performance.
Tip 7: Leverage Concurrency Mechanisms: Implement proper concurrency mechanisms to handle multiple concurrent requests, ensuring accurate calculations in high-traffic scenarios.
By following these tips, you can develop robust and efficient discount percentage calculation mechanisms in ASP.NET C#, enhancing the user experience and ensuring accurate financial transactions.
In the concluding section, we will discuss advanced techniques and best practices to further optimize and enhance your discount percentage calculation capabilities.
Conclusion
Throughout this article, we have delved into the intricacies of “how to calculate discount percentage in asp.net c#”. We explored the mathematical formula, delved into data types and rounding techniques, and highlighted the importance of error handling, performance optimization, and unit testing. By mastering these aspects, developers can ensure accurate and reliable discount percentage calculations in their applications.
Two key points to remember are: 1) Understanding the formula and data types is essential for precise calculations. 2) Error handling and performance optimization techniques are crucial for robust and efficient code. These elements are interconnected and contribute to the overall quality and user experience of the application.
As you embark on implementing discount percentage calculations in ASP.NET C#, remember the significance of accuracy, efficiency, and maintainability. By following the insights and best practices outlined in this article, you can develop robust and reliable applications that empower businesses to offer compelling discounts and enhance customer satisfaction.
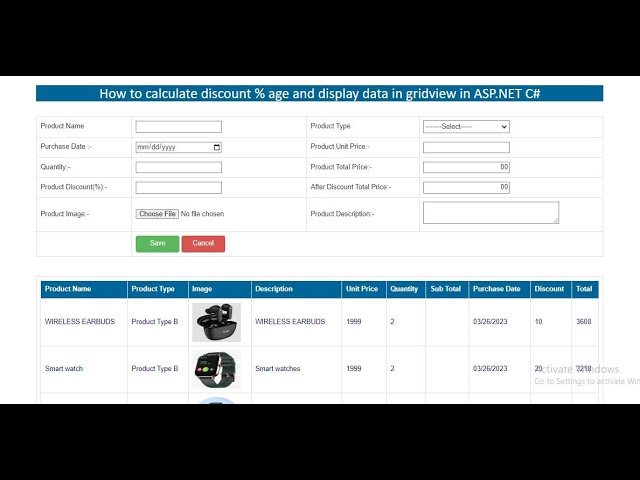