Calculating discount percentages in PHP is a fundamental skill for developers working with e-commerce or financial applications. It involves determining the reduced price of an item or service based on a given discount rate.
Understanding how to calculate discount percentages in PHP is crucial for various reasons. It enables developers to implement accurate pricing calculations, display discounts to customers, and create automated processes for managing sales and promotions. Historically, this calculation has played a vital role in the evolution of e-commerce, allowing businesses to offer flexible discounts and attract customers.
This article will delve into the detailed steps involved in calculating discount percentages in PHP, providing clear examples and practical guidance to help developers implement this functionality in their applications.
How to Calculate Discount Percentage in PHP
Calculating discount percentages in PHP is a fundamental skill for developers working with e-commerce or financial applications. It involves determining the reduced price of an item or service based on a given discount rate.
- Formula: The mathematical formula used to calculate the discount percentage.
- Variables: The input values required for the calculation, such as original price and discount rate.
- Data Types: The specific data types used to represent the input values and the result.
- Functions: PHP functions that can be used to perform the calculation, such as the number_format() function.
- Syntax: The correct syntax for writing the code to calculate the discount percentage.
- Examples: Practical examples demonstrating how to use the formula and functions to calculate discount percentages.
- Applications: The various applications of discount percentage calculation in real-world scenarios.
- Optimization: Techniques to optimize the code for better performance and efficiency.
- Error Handling: Strategies for handling errors and exceptions that may occur during the calculation.
These key aspects provide a comprehensive understanding of how to calculate discount percentages in PHP, enabling developers to implement this functionality accurately and efficiently in their applications.
Formula
The mathematical formula used to calculate the discount percentage is the cornerstone of understanding how to calculate discount percentage in PHP. It provides the mathematical foundation for determining the reduced price of an item or service based on a given discount rate.
- Percentage Discount: The most common type of discount, represented as a percentage of the original price, e.g., 20% discount.
- Fixed Amount Discount: A specific amount of money deducted from the original price, e.g., $10 off.
- Tiered Discount: Discounts that vary based on the quantity purchased or the total amount of the order, e.g., 10% off for orders over $100.
- Combined Discounts: A combination of different discount types, e.g., 15% off and an additional $5 for orders over $50.
These formulas and their variations form the basis for calculating discount percentages in PHP, enabling developers to implement accurate pricing calculations, display discounts to customers, and create automated processes for managing sales and promotions.
Variables
In the context of calculating discount percentages in PHP, variables play a crucial role as the input values for the calculation. These variables represent the original price of the item or service, as well as the discount rate or amount.
- Original Price: The original or full price of the item or service before any discount is applied. This is a mandatory input value.
- Discount Rate/Percentage: The discount rate or percentage that is to be applied to the original price to determine the reduced price. This can be a numeric value or a percentage represented as a decimal.
- Discount Amount: In cases where a fixed amount discount is applied, this variable represents the specific amount of money to be deducted from the original price.
- Discount Type: In scenarios involving tiered or combined discounts, this variable indicates the type of discount being applied, such as percentage discount, fixed amount discount, or a combination thereof.
Understanding the significance and proper usage of these input variables is essential for accurate calculation of discount percentages in PHP. They serve as the foundation for determining the reduced price, displaying discounts to customers, and managing sales and promotions effectively.
Data Types
In the context of PHP programming, data types play a pivotal role in representing the input values (original price, discount rate/amount) and the resulting discounted price accurately.
- Integer: Whole numbers without decimal points, commonly used for representing the original price and discount amount when they are whole numbers.
- Float: Numbers with decimal points, suitable for representing the original price and discount rate/amount when they involve fractional parts.
- String: Textual data, used in scenarios where the discount rate/percentage is represented as a string (e.g., “20% off”).
- Boolean: Logical values (true/false), potentially used in conditional statements involving discount eligibility or specific discount scenarios.
Understanding and using the appropriate data types ensures accurate calculations, prevents errors, and facilitates seamless integration with other components of the PHP application.
Functions
In the context of calculating discount percentages in PHP, leveraging the appropriate PHP functions becomes crucial. These functions provide pre-built functionality to perform mathematical operations, format the output, and handle edge cases.
- Core Mathematical Functions: Functions like round(), ceil(), and floor() assist in rounding the results to the desired precision, ensuring accurate discount calculations.
- Formatting Functions: The number_format() function enables developers to format the discounted price with appropriate decimal places and currency symbols, enhancing readability for end-users.
- Conditional Functions: Functions like min() and max() can be employed to enforce minimum or maximum discount limits, ensuring that the discounted price falls within specified boundaries.
- Error Handling Functions: In the event of invalid inputs or unexpected scenarios, functions like is_numeric() and trigger_error() enable developers to handle errors gracefully, providing a better user experience and facilitating debugging.
Harnessing these PHP functions empowers developers to perform discount percentage calculations efficiently, handle various scenarios, and deliver a robust and user-friendly application.
Syntax
In the realm of programming, syntax holds paramount importance, serving as the foundation upon which functional code is built. In the context of “how to calculate discount percentage in php,” syntax plays a pivotal role, dictating the precise arrangement of code elements to achieve the desired outcome.
A correctly structured syntax ensures that the code is not only executable by the PHP interpreter but also readable and maintainable by fellow developers. It involves adhering to specific rules and conventions, such as proper indentation, variable naming, and statement termination. Without a valid syntax, the code will fail to compile or produce unexpected results.
Real-life examples abound to illustrate the significance of syntax in discount percentage calculation. Consider a simple PHP script that calculates the discounted price of an item based on a given percentage:
$original_price = 100;$discount_percentage = 20;$discount_amount = $original_price * $discount_percentage / 100;$discounted_price = $original_price - $discount_amount;echo "Discounted Price: $discounted_price";
In this example, the syntax dictates the order of operations, the use of variables, and the mathematical formula for calculating the discount. Any deviation from the correct syntax, such as a missing semicolon or incorrect variable name, would render the code non-functional.
Understanding the syntax enables developers to write robust and efficient code, troubleshoot errors effectively, and adapt to changing requirements. It empowers them to create reusable functions and libraries, promoting code sharing and collaboration within the development team.
Examples
In the realm of “how to calculate discount percentage in php,” examples serve as a cornerstone, bridging the gap between theoretical understanding and practical implementation. These examples provide step-by-step demonstrations, utilizing real-world scenarios and code snippets, to illustrate the application of formulas and functions in calculating discount percentages.
Their importance lies in their ability to reinforce concepts, clarify complex topics, and foster a deeper comprehension of the subject matter. By working through concrete examples, learners can visualize the practical implications of the formulas and functions, empowering them to confidently apply these techniques in their own projects or assignments.
For instance, an example might showcase the calculation of a discounted price for an item with a given original price and discount rate. The example would walk through the process of applying the formula, using specific values and demonstrating the use of PHP functions like round() to format the result. This hands-on approach enables learners to grasp the practical significance of the concepts and apply them effectively.
In summary, examples play a crucial role in “how to calculate discount percentage in php” by providing practical illustrations, clarifying complex topics, and fostering a deeper understanding of the subject matter. They serve as a valuable resource for learners and developers alike, equipping them with the skills and confidence to implement these techniques in their own projects.
Applications
In the realm of “how to calculate discount percentage in php,” understanding the practical applications of discount percentage calculation holds paramount importance. It establishes the relevance and significance of the topic, demonstrating how this knowledge extends beyond theoretical concepts and into the practical world.
Discount percentage calculation finds its applications in diverse real-world scenarios, ranging from e-commerce platforms to inventory management systems. In e-commerce, it enables businesses to offer discounts and promotions to attract customers, boost sales, and clear inventory. For instance, a 20% discount on a product with an original price of $100 translates to a discounted price of $80, making it more appealing to budget-conscious shoppers.
In inventory management, discount percentage calculation aids in determining the appropriate markdown prices for products approaching their expiration dates or when there is excess stock. By calculating the discount percentage, businesses can effectively reduce losses and optimize their inventory levels. Moreover, it finds applications in financial institutions, where it is used to calculate interest rates, loan repayments, and other financial transactions.
In summary, understanding discount percentage calculation in php equips developers with the skills to implement these techniques in a variety of real-world applications. It empowers them to create dynamic pricing strategies, optimize inventory management, and contribute to the development of robust financial systems.
Optimization
In the realm of “how to calculate discount percentage in php,” optimization techniques play a vital role in enhancing the performance and efficiency of the code. These techniques aim to minimize resource consumption, reduce execution time, and improve overall responsiveness.
- Caching:
Caching involves storing frequently used data or calculations in memory to avoid repetitive processing. In the context of discount percentage calculation, caching the results for commonly used discount rates can significantly improve performance.
- Data Type Optimization:
Choosing the appropriate data types for variables can impact performance. Using integers instead of floats for whole numbers and optimizing the precision of floating-point calculations can reduce memory usage and improve speed.
- Algorithm Selection:
Selecting the most efficient algorithm for the task at hand is crucial. For example, using the round() function instead of a custom rounding algorithm can improve performance, as it is optimized for this specific task.
- Code Profiling:
Code profiling tools can identify bottlenecks and inefficiencies in the code. By analyzing the execution time and resource consumption of different code sections, developers can pinpoint areas for optimization.
By applying these optimization techniques, developers can create code that is not only accurate but also efficient and performant. This is particularly important in e-commerce applications where discounts are applied to numerous items and performance is crucial for a seamless user experience.
Error Handling
Within the context of “how to calculate discount percentage in PHP”, error handling plays a crucial role in ensuring the reliability and robustness of the code. Errors and exceptions can arise due to various reasons, such as invalid input data, incorrect mathematical operations, or system-level issues. A well-defined error handling strategy enables developers to manage these situations gracefully, preventing the code from crashing and providing meaningful feedback to users.
One common approach to error handling involves using try-catch blocks. This allows developers to define specific actions to be taken when an error or exception occurs. For instance, if the discount percentage entered by a user exceeds 100%, the code can catch this error and display an appropriate error message, allowing the user to correct the input.
Additionally, error logging is a valuable technique for debugging and troubleshooting purposes. By logging error messages and relevant details, developers can easily identify the source of the problem and implement appropriate fixes. This information is particularly useful when dealing with intermittent or complex errors that may be difficult to reproduce.
In summary, error handling is a critical component of “how to calculate discount percentage in PHP” as it ensures the stability and reliability of the code. By implementing effective error handling strategies, developers can handle errors and exceptions gracefully, provide informative feedback to users, and facilitate efficient debugging processes.
Frequently Asked Questions
This FAQ section aims to address common questions and clarify aspects related to “how to calculate discount percentage in PHP”.
Question 1: What is the formula for calculating discount percentage?
Answer: The formula is: Discount Percentage = (Discount Amount / Original Price) x 100.
Question 2: How to handle negative values for discount percentage or original price?
Answer: Negative values should be avoided as they result in invalid calculations. Ensure that both the discount percentage and original price are non-negative.
Question 3: What is the difference between discount percentage and discount amount?
Answer: Discount percentage is expressed as a percentage, while discount amount is the actual value deducted from the original price.
Question 4: How to calculate discounts for multiple items with different discount percentages?
Answer: Calculate the discount for each item individually using the formula and then sum up the discounted prices.
Question 5: What are some common errors to avoid when calculating discount percentages?
Answer: Common errors include using incorrect data types, dividing by zero, and exceeding 100% discount.
Question 6: How to optimize the code for calculating discount percentages?
Answer: Optimization techniques include using caching, choosing appropriate data types, and leveraging efficient algorithms.
These FAQs provide key insights into the concepts and techniques related to discount percentage calculation in PHP.
In the next section, we will delve deeper into the practical implementation of these concepts, exploring code examples and real-world applications.
Tips on Discount Percentage Calculation in PHP
This section provides practical tips to enhance your understanding and implementation of discount percentage calculation in PHP.
Tip 1: Use Named Constants: Define named constants for common discount rates, such as “STANDARD_DISCOUNT” and “CLEARANCE_DISCOUNT”, to improve code readability and maintainability.
Tip 2: Validate Input: Always validate user input to ensure that discount percentages and original prices are valid and within expected ranges.
Tip 3: Leverage Built-in Functions: Utilize PHP’s built-in functions, such as number_format() and round(), for formatting and rounding discount amounts.
Tip 4: Consider Tiered Discounts: Implement tiered discounts based on purchase quantity or total amount to encourage bulk purchases and increase customer loyalty.
Tip 5: Optimize for Performance: Cache frequently used discount rates and employ efficient algorithms to minimize calculation time, especially for high-volume systems.
Tip 6: Handle Errors Gracefully: Implement comprehensive error handling to gracefully handle invalid inputs, calculation errors, and unexpected scenarios.
Tip 7: Use Unit Tests: Write unit tests to verify the accuracy and robustness of your discount calculation code, covering various scenarios and edge cases.
By incorporating these tips, you can write reliable and efficient code for calculating discount percentages in PHP, enhancing the functionality and user experience of your applications.
In the next section, we will explore some real-world examples of discount percentage calculation, demonstrating its practical applications in various domains.
Conclusion
Throughout this article, we have delved into the intricacies of “how to calculate discount percentage in PHP.” We explored the mathematical formula, data types, functions, syntax, and various applications of discount percentage calculation.
Key points to remember include:
- Discount percentage calculation involves applying the formula: Discount Percentage = (Discount Amount / Original Price) x 100.
- Variables involved are original price, discount rate/amount, and discount type, with appropriate data types (integer, float, string, boolean) used for representation.
- PHP functions like number_format() and round() assist in formatting and rounding the discounted price, ensuring accuracy and readability.
Understanding these concepts and implementing them effectively enables developers to create robust e-commerce systems, optimize inventory management, and enhance financial applications. Discount percentage calculation is a fundamental aspect of pricing strategies, sales promotions, and customer loyalty programs, making it a valuable skill for web developers and programmers.
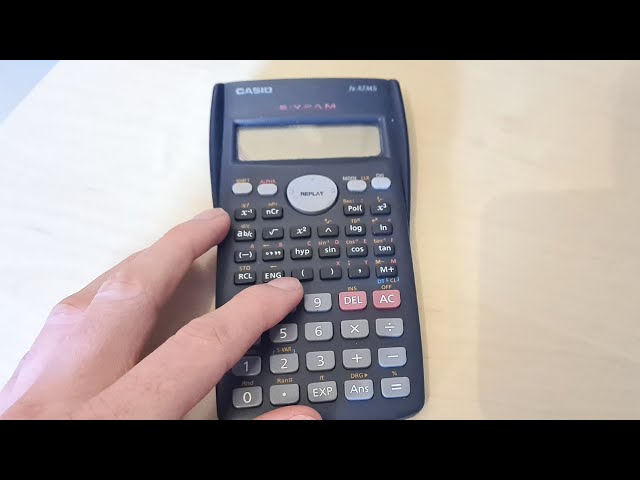