Calculating discounts in Java is a crucial task in various domains like e-commerce and finance. It involves determining the reduced price of an item or service based on a specified discount percentage.
Understanding how to calculate discounts in Java is essential for developers to create efficient and accurate applications. Historically, the concept of discounts has evolved over time, with businesses employing various strategies to incentivize purchases and manage inventory levels.
In this article, we will explore the fundamentals of calculating discounts in Java, covering the relevant mathematical formulas, code snippets, and best practices. By delving into these concepts, we aim to empower Java developers with the knowledge and techniques to effectively handle discount calculations in their applications.
How to Calculate Discount in Java
Understanding the essential aspects of calculating discounts in Java is crucial for effective application development.
- Mathematical Formulas
- Code Snippets
- Best Practices
- Percentage Calculation
- Amount-Based Discounts
- Multiple Discount Scenarios
- Data Types and Precision
- Exception Handling
- Testing and Validation
These aspects encompass the core concepts, techniques, and considerations involved in discount calculations. By exploring these elements, developers can gain a comprehensive understanding of how to accurately and efficiently implement discount functionality in their Java applications.
Mathematical Formulas
Mathematical formulas serve as the cornerstone of calculating discounts in Java. They provide a systematic approach to determining the discounted price based on the original price and the discount percentage. Understanding these formulas is essential for developers to implement accurate and efficient discount calculations in their applications.
- Percentage Calculation
The most fundamental formula involves calculating the discount as a percentage of the original price. This is achieved by multiplying the original price by the discount percentage expressed as a decimal.
- Amount-Based Discounts
In some cases, discounts may be specified as a fixed amount rather than a percentage. To calculate the discounted price in such scenarios, the discount amount is directly subtracted from the original price.
- Multiple Discount Scenarios
Real-world scenarios often involve applying multiple discounts or combining different discount types. Mathematical formulas provide a framework for handling such complexities by allowing developers to define and apply discount rules in a structured manner.
- Data Types and Precision
When working with monetary values in Java, choosing the appropriate data types and considering precision is crucial. Mathematical formulas should be adapted to handle different data types, such as integers, floating-point numbers, or BigDecimal, to ensure accurate calculations.
Grasping these mathematical formulas empowers Java developers to confidently tackle discount calculations in their applications. By leveraging these formulas, they can ensure precise and reliable calculations that meet the business requirements and enhance the user experience.
Code Snippets
Code snippets play a pivotal role in understanding how to calculate discounts in Java. They provide practical and executable examples that illustrate the implementation of discount calculations in Java code. By examining code snippets, developers can gain insights into the syntax, data structures, and algorithms involved in discount calculations.
Code snippets are critical components of tutorials and documentation on discount calculations in Java. They allow readers to visualize the concepts and apply them directly in their own code. Real-life examples of code snippets include calculating discounts based on a percentage, applying multiple discounts, and handling special cases such as negative discounts or invalid inputs.
Understanding code snippets empowers Java developers to implement discount functionality in their applications with confidence and efficiency. By leveraging code snippets as building blocks, developers can explore different approaches, experiment with different scenarios, and ultimately create robust and maintainable code.
Best Practices
In the context of discount calculations in Java, adhering to best practices is crucial for developing robust, efficient, and maintainable code. These best practices encompass various facets, including:
- Clear and Consistent Naming
Utilizing clear and consistent variable, method, and class names enhances code readability and comprehension. This practice aids in understanding the purpose and relationships between different components involved in discount calculations.
- Exception Handling
Anticipating and handling potential exceptions that may arise during discount calculations is essential. This involves implementing robust error-handling mechanisms to gracefully handle invalid inputs, data inconsistencies, or system failures.
- Testing and Validation
Thorough testing and validation of discount calculations ensure accuracy and reliability. Unit tests can be employed to verify individual components, while integration tests assess the interactions between different modules involved in discount processing.
- Performance Optimization
Optimizing the performance of discount calculations is crucial for applications handling a high volume of transactions. Techniques such as caching and indexing can be implemented to minimize latency and improve overall responsiveness.
By adhering to these best practices, Java developers can create discount calculation functionality that is not only accurate and efficient but also maintainable and scalable for evolving business requirements.
Percentage Calculation
Percentage calculation forms a crucial aspect of “how to calculate discount in java.” It involves determining the discount amount as a percentage of the original price, often represented as a decimal value. Understanding percentage calculation is foundational for developing accurate and efficient Java applications that handle discounts.
- Formula and Syntax
The core formula for calculating the discount as a percentage is discount = original_price * discount_rate, where discount_rate is expressed as a decimal (e.g., 0.1 for a 10% discount).
- Real-World Examples
In e-commerce, percentage discounts are commonly used to offer promotions and seasonal sales. For instance, a 20% discount on a $100 item translates to a discount of $20.
- Precision and Data Types
When working with monetary values and percentage calculations in Java, choosing appropriate data types and considering precision is essential. Java offers data types like int, float, and BigDecimal, each with its own characteristics for handling precision and scale.
- Error Handling
Robust error handling is crucial to manage invalid inputs and exceptional conditions during percentage calculations. This includes handling negative values or invalid discount rates to ensure the application’s stability and reliability.
In summary, understanding percentage calculation is vital for accurate discount computations in Java. By leveraging these concepts and adhering to best practices, developers can implement effective and reliable discount calculation functionality in their applications, enhancing the user experience and ensuring accurate financial transactions.
Amount-Based Discounts
In the broader context of “how to calculate discount in java”, “Amount-Based Discounts” represent a distinct strategy for reducing the price of goods or services. Unlike percentage-based discounts, which are calculated as a proportion of the original price, amount-based discounts offer a fixed reduction in monetary value.
- Fixed Amount Discounts
The most straightforward form of amount-based discounts involves deducting a predefined monetary amount from the original price, regardless of the item’s value. For example, a $10 discount on a $50 product.
- Tiered Discounts
This approach offers varying discount amounts based on the quantity or value of items purchased. For instance, a 10% discount for purchases over $100 and a 15% discount for purchases over $200.
- Volume Discounts
Volume discounts incentivize bulk purchases by offering a greater discount for larger quantities. For example, a 5% discount for orders of 10 units and a 10% discount for orders of 20 or more units.
- Loyalty Discounts
Amount-based discounts can be used to reward customer loyalty. For instance, a $5 discount on every fifth purchase or a 10% discount for members of a loyalty program.
Understanding the nuances of amount-based discounts is crucial for effective implementation in Java applications. These discounts offer flexibility in pricing strategies, allowing businesses to tailor discounts to specific customer segments, encourage bulk purchases, and foster customer loyalty. By incorporating these concepts into their code, Java developers can create sophisticated and adaptable discount calculation functionality.
Multiple Discount Scenarios
In the realm of “how to calculate discount in java,” “Multiple Discount Scenarios” arise when discounts are applied in various combinations or sequences. Understanding these scenarios is crucial for accurate calculations and effective implementation of discount strategies.
- Sequential Discounts
Discounts can be applied sequentially, where one discount is applied after another. For example, a 10% discount followed by a 5% discount on the discounted price.
- Combined Discounts
Multiple discounts can be combined, offering a greater overall discount. For instance, a 15% discount on all items combined with a buy-one-get-one-free promotion.
- Tiered Discounts
Discounts can be structured in tiers based on purchase quantity or value, leading to multiple discount rates. For example, a 5% discount for purchases over $50 and a 10% discount for purchases over $100.
- Loyalty Discounts
Loyalty programs often offer tiered or combined discounts to reward repeat customers. These discounts can vary based on membership level or purchase history.
Handling multiple discount scenarios requires careful consideration of the order of application, eligibility criteria, and potential overlaps. Java developers must design robust code that accurately calculates discounts based on the defined rules and scenarios. By understanding these complexities, developers can create flexible and adaptable discount calculation functionality that caters to diverse business requirements.
Data Types and Precision
In the context of “how to calculate discount in java,” “Data Types and Precision” play a critical role in ensuring accurate and reliable discount calculations. Data types define the type of values that can be stored in a variable, while precision refers to the accuracy or level of detail in representing those values. Choosing appropriate data types and maintaining precision are essential for handling monetary values and discount calculations effectively.
For example, if a discount is represented as a percentage, using the data type “float” may result in precision errors due to its limited decimal precision. Instead, using “double” or “BigDecimal” data types provides higher precision and accuracy in calculations, especially when dealing with small or fractional discount values.
Understanding the relationship between data types and precision is crucial for Java developers to make informed choices and avoid potential errors or inconsistencies in discount calculations. By selecting the correct data types and considering precision, developers can ensure that discount calculations are accurate, reliable, and meet the requirements of the specific application.
Exception Handling
Exception handling is a crucial aspect of “how to calculate discount in java” as it enables developers to anticipate and manage potential errors or exceptional conditions that may arise during discount calculations. Without proper exception handling, these errors can lead to unexpected behavior, incorrect results, or even system failures.
A common example of an exception in discount calculations is attempting to apply a discount to an invalid input, such as a negative price or an invalid discount rate. By implementing exception handling, developers can gracefully handle such errors, providing informative error messages and taking appropriate corrective actions, such as rejecting the input or providing a default discount value.
Exception handling is particularly important in scenarios where discount calculations are performed in real-time or integrated with other systems. Proper handling of exceptions ensures that the application remains stable and responsive, preventing cascading errors or data inconsistencies that could impact the overall functionality or user experience.
In summary, exception handling plays a critical role in “how to calculate discount in java” by enabling developers to anticipate and manage errors, ensuring the accuracy, reliability, and stability of the discount calculation process. Understanding and implementing exception handling techniques is essential for developing robust and user-friendly Java applications that handle discounts effectively.
Testing and Validation
Testing and Validation are crucial aspects of “how to calculate discount in java” as they ensure the correctness, reliability, and accuracy of the discount calculation process. Through a combination of techniques, developers can verify that the code functions as expected and produces accurate results under different conditions.
- Unit Testing
Unit testing involves testing individual components or functions of the discount calculation logic in isolation. By isolating specific parts of the code, developers can thoroughly test their behavior and identify potential errors or inconsistencies.
- Integration Testing
Integration testing focuses on testing how different components of the discount calculation system work together. It ensures that the components interact correctly and that the overall system functions as intended.
- Regression Testing
Regression testing is performed after code changes or updates to ensure that existing functionality remains intact. It helps identify any unintended consequences or errors introduced by the changes.
- Performance Testing
Performance testing evaluates the efficiency and scalability of the discount calculation system under varying loads or conditions. It helps identify potential bottlenecks or performance issues that may impact the user experience or system stability.
By implementing comprehensive testing and validation strategies, developers can gain confidence in their discount calculation code, ensuring that it delivers accurate results, handles exceptional conditions gracefully, and meets the performance requirements of the application. This ultimately enhances the overall reliability and user satisfaction of the system.
Frequently Asked Questions
This section addresses common questions and concerns regarding discount calculations in Java. These FAQs aim to clarify key concepts and provide additional insights to enhance your understanding.
Question 1: What are the different methods for calculating discounts in Java?
There are two primary methods for calculating discounts in Java: percentage-based discounts and amount-based discounts. Percentage-based discounts are calculated as a percentage of the original price, while amount-based discounts involve deducting a fixed amount from the original price.
Question 2: How do I handle invalid inputs or exceptional conditions during discount calculations?
It is crucial to implement proper exception handling to manage invalid inputs and exceptional conditions. This involves anticipating potential errors and implementing code to handle them gracefully, providing informative error messages and taking appropriate actions.
Question 3: What are the best practices for ensuring accurate and reliable discount calculations?
Adhering to best practices such as using appropriate data types, maintaining precision, and implementing thorough testing and validation strategies is essential for ensuring accurate and reliable discount calculations.
Question 4: How can I optimize the performance of discount calculation code?
To optimize performance, consider techniques such as caching or indexing to minimize latency and improve responsiveness, especially when handling large volumes of discount calculations.
Question 5: What are some common pitfalls or challenges in discount calculations?
Common pitfalls include handling multiple discount scenarios, dealing with data precision issues, and managing exceptional conditions effectively. Understanding these challenges and implementing robust solutions is crucial for developing efficient and reliable discount calculation code.
Question 6: How can I extend or customize the discount calculation functionality in Java?
Java provides flexibility in extending and customizing discount calculation functionality. You can define custom discount rules, implement tiered or volume-based discounts, and integrate with external systems or databases to enhance the capabilities of your discount calculation code.
These FAQs provide a concise overview of key considerations and best practices for discount calculations in Java. By understanding these concepts and applying them effectively, you can develop robust and reliable code that meets the requirements of your project.
As we delve deeper into the topic, the subsequent section will explore advanced techniques and considerations for complex discount scenarios, such as handling multiple discount types, managing hierarchical discounts, and integrating with external data sources.
Tips for Effective Discount Calculations in Java
This section provides practical tips and recommendations to enhance the effectiveness and accuracy of your discount calculation code in Java.
Tip 1: Utilize Appropriate Data Structures
Choose suitable data structures, such as BigDecimal, to handle monetary values and maintain precision in calculations.Tip 2: Implement Robust Exception Handling
Anticipate and handle potential errors or invalid inputs gracefully to maintain the stability and reliability of your code.Tip 3: Consider Multiple Discount Scenarios
Handle complex scenarios involving sequential, combined, or tiered discounts to ensure accurate calculations in various situations.Tip 4: Optimize Performance for Scalability
Employ techniques like caching or indexing to improve the efficiency of discount calculations, especially when dealing with large volumes.Tip 5: Leverage Java’s Built-In Math Library
Utilize Java’s Math class for operations like rounding, absolute value calculation, and more to enhance the precision and accuracy of your code.Tip 6: Perform Thorough Testing and Validation
Implement comprehensive testing strategies, including unit testing, integration testing, and performance testing, to ensure the correctness and reliability of your discount calculation logic.Tip 7: Adhere to Coding Standards and Best Practices
Follow established coding standards and best practices to maintain code quality, readability, and maintainability.Tip 8: Seek External Resources and Community Support
Explore online resources, forums, and communities to learn from others, stay updated on best practices, and seek assistance when needed.
By incorporating these tips into your Java code, you can develop more robust, efficient, and accurate discount calculation functionality that meets the demands of your application.
In the concluding section, we will delve into advanced techniques and considerations for complex discount scenarios, further enhancing your understanding and empowering you to handle even the most challenging discount calculation requirements.
Conclusion
This article has provided a comprehensive exploration of “how to calculate discount in java,” delving into the various aspects, techniques, and considerations involved in accurate and efficient discount calculations. Key ideas and findings include the understanding of different discount types (percentage-based and amount-based), handling multiple discount scenarios, ensuring data precision and exception handling, and the importance of testing and validation for reliable results.
To recap the main points, firstly, selecting the appropriate discount calculation method based on the business requirement is crucial. Secondly, managing complex discount scenarios, such as combining multiple discounts or handling tiered discounts, requires careful consideration and robust code implementation. Lastly, adhering to best practices, including proper data handling, exception handling, and testing, is essential for developing high-quality discount calculation code.
In essence, mastering the art of discount calculations in Java empowers developers to create sophisticated e-commerce and financial applications that can effectively manage discounts, enhance customer satisfaction, and optimize revenue generation. As the world of e-commerce continues to evolve, staying abreast of the latest techniques and best practices in discount calculations will remain a valuable asset for Java developers.
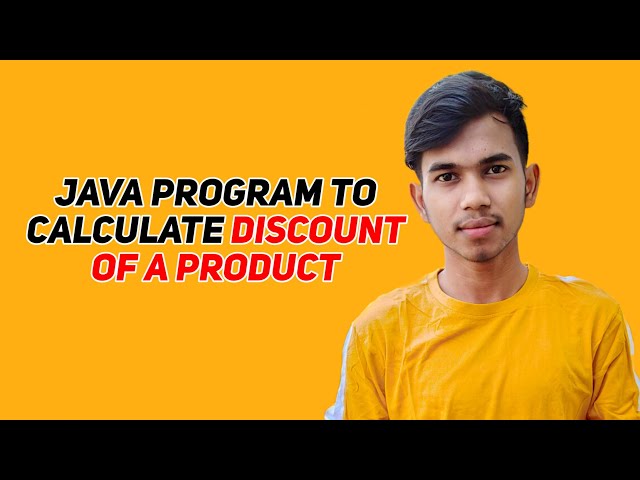