Calculating discounts in Python involves applying mathematical operations to reduce the original price of an item. For example, a product with a regular price of $100 could be discounted by 20%, resulting in a reduced price of $80. Understanding how to calculate discounts in Python has numerous benefits and is crucial in various industries, such as retail, e-commerce, and finance.
To calculate discounts in Python, you can use the built-in math library. The formula for calculating the discount is: discount = price * discount_rate, where price is the original price and discount_rate is the percentage of the discount expressed as a decimal (e.g., 20% would be 0.20). By incorporating this formula into Python code, you can automate the discount calculation process.
In this article, we will delve into the specifics of calculating discounts in Python. We will provide detailed explanations of the necessary concepts, step-by-step instructions, and code examples to guide you through the process effectively.
How to Calculate Discount in Python
Calculating discounts in Python is a crucial aspect of many applications, such as retail, e-commerce, and finance. It involves applying mathematical operations to reduce the original price of an item, and understanding the key aspects of this process is essential.
- Formula: discount = price * discount_rate
- Built-in Functions: math library
- Data Types: float, decimal
- Variable Names: price, discount_rate, discount
- Error Handling: invalid inputs, negative values
- Code Optimization: efficiency, readability
- Testing: unit tests, integration tests
- Documentation: clear instructions, examples
These key aspects provide a comprehensive understanding of how to calculate discounts in Python. The formula is the foundation for performing the calculation, and the built-in functions simplify the process. Data types and variable names ensure accuracy and readability, while error handling prevents unexpected behavior. Code optimization enhances performance, and testing ensures reliability. Finally, documentation guides users and facilitates maintenance.
Formula
The formula discount = price discount_rate is central to calculating discounts in Python. It provides a concise mathematical expression for determining the discounted price of an item. Understanding the components and implications of this formula is crucial for effective discount calculations.
- Price: The original price of the item before any discount is applied.
- Discount Rate: The percentage of the discount expressed as a decimal. For example, a 20% discount would be represented as 0.20.
- Discount: The amount of reduction applied to the price. Calculated by multiplying the price by the discount rate.
- Relationships: This formula highlights the direct relationship between the discount, price, and discount rate. Changes in any of these variables will impact the final discounted price.
Comprehending these facets empowers developers to accurately calculate discounts in Python. This formula forms the basis for implementing discount functionality in various applications, ensuring precise and reliable results.
Built-in Functions
Within the context of calculating discounts in Python, the math library is an indispensable tool that provides a range of functions for performing mathematical operations. Its comprehensive functionality empowers developers to implement discount calculations with precision and efficiency.
- Mathematical Functions: The math library offers a plethora of mathematical functions such as ceil(), floor(), and pow(), which can be leveraged for advanced discount calculations involving rounding, exponents, and other complex operations.
- Constants: The library provides access to mathematical constants such as pi and e, which can be useful in specific discount calculations or as part of more elaborate formulas.
- Trigonometric Functions: While not directly related to discount calculations, the math library’s trigonometric functions (e.g., sin(), cos(), tan()) can be employed in specialized scenarios where angles or periodic patterns are involved.
- Error Handling: The math library includes functions for handling errors and exceptions that may arise during discount calculations, ensuring program stability and robustness.
These facets of the math library underscore its significance in the realm of discount calculations in Python. By harnessing its capabilities, developers can craft precise and versatile discount calculation logic that meets the demands of various applications.
Data Types
In the context of calculating discounts in Python, the choice of data types, particularly float and decimal, plays a critical role in ensuring accurate and reliable results. These data types are specifically designed to represent numerical values, with distinct characteristics that impact the precision and behavior of discount calculations.
Float, short for floating-point, is a data type that approximates real numbers using a binary format. It offers a wide range of values and a large exponent range, making it suitable for general-purpose calculations. However, floats have limited precision, which can introduce rounding errors, especially when dealing with monetary values or calculations involving small differences.
Decimal, on the other hand, is a data type specifically designed for precise decimal calculations. It uses a fixed-point representation, ensuring exact results even for complex operations. Decimals are particularly valuable in financial applications, where accurate calculations and avoidance of rounding errors are crucial. For discount calculations involving currency values or precise percentage adjustments, decimals provide a reliable and accurate solution.
Understanding the distinction between float and decimal data types and their implications on discount calculations is essential for Python developers. Choosing the appropriate data type based on the specific requirements of the application ensures accurate and reliable results, preventing potential errors or unexpected behavior in discount calculations.
Variable Names
In the context of calculating discounts in Python, the aptly chosen variable names price, discount_rate, and discount play a pivotal role in enhancing code readability, maintainability, and overall clarity. These variable names align precisely with the concepts they represent, fostering an intuitive understanding of the code’s functionality.
The variable price denotes the original price of the item before any discount is applied, setting the baseline for the calculation. Discount_rate, expressed as a decimal, signifies the percentage of the discount being offered, directly influencing the discounted price. Finally, the aptly named discount variable stores the calculated amount of reduction applied to the price.
Real-life examples abound, consider a retail application where a product with a regular price of $100 is offered a 20% discount. Using the formula discount = price * discount_rate, the code would assign the values price = 100 and discount_rate = 0.20, resulting in a discount of $20. This clear and self-explanatory variable naming convention enables developers to grasp the code’s intent swiftly, promoting efficient code comprehension and modification.
Understanding the connection between these variable names and discount calculation in Python is essential for several reasons. First, it facilitates seamless code comprehension, allowing developers to decipher the code’s purpose and functionality without hindrance. Second, it fosters code maintainability, as well-named variables reduce the likelihood of errors and make code modifications more straightforward. Finally, it promotes a consistent and standardized approach to discount calculations, ensuring code uniformity and reducing the risk of errors due to inconsistent variable naming conventions.
Error Handling
In the context of calculating discounts in Python, error handling plays a pivotal role in ensuring the robustness and reliability of the code. Invalid inputs and negative values pose potential challenges that must be addressed to prevent unexpected behavior and incorrect results.
Invalid inputs, such as non-numeric characters or incorrect data formats, can lead to errors during the calculation process. For instance, if a user enters a letter instead of a number for the discount rate, the code may fail to perform the calculation correctly. Similarly, negative values for the price or discount rate can result in nonsensical or erroneous results, as discounts are typically applied to positive values.
To mitigate these issues, robust error handling mechanisms should be implemented to validate inputs and handle edge cases gracefully. This can involve checking for invalid data types, ensuring that values are within expected ranges, and providing informative error messages to guide users in correcting their inputs. By incorporating comprehensive error handling, developers can enhance the user experience and prevent incorrect calculations.
In summary, error handling is a critical aspect of calculating discounts in Python. By addressing invalid inputs and negative values, developers can create more robust and reliable code. This not only improves the user experience but also ensures the accuracy and integrity of the calculated discounts.
Code Optimization
In the context of calculating discounts in Python, code optimization plays a vital role, with efficiency and readability being key considerations. Optimized code ensures swift execution and crystal-clear comprehension, both essential for effective discount calculations.
Code efficiency directly impacts the performance of discount calculations, particularly in situations involving a large volume of transactions or complex calculations. Well-optimized code minimizes resource consumption, reducing execution time and enhancing overall system responsiveness. This is particularly important in real-time applications or environments where rapid response is crucial.
Readability, on the other hand, contributes to the maintainability and longevity of the codebase. Well-structured and well-documented code is easier to comprehend and modify, reducing the likelihood of errors and facilitating future enhancements. This becomes increasingly important as the codebase evolves and multiple developers collaborate on the project.
To illustrate, consider a Python function that calculates discounts based on a given price and discount rate. If the code is poorly optimized, it may perform unnecessary calculations or use inefficient algorithms, resulting in slower execution times. On the other hand, optimized code would employ efficient data structures and algorithms, minimizing resource consumption and improving performance.
Understanding the connection between code optimization and discount calculations in Python empowers developers to craft efficient and readable code. This not only enhances the performance and maintainability of their applications but also promotes a deeper understanding of the underlying principles, enabling them to tackle more complex challenges in the future.
Testing
In the domain of discount calculations in Python, testing plays a pivotal role in ensuring the accuracy, reliability, and robustness of the code. Unit tests and integration tests serve as essential components of the testing strategy, each with distinct purposes and contributions to the overall quality of the codebase.
Unit tests focus on testing individual functions or modules in isolation, examining their behavior under various inputs and conditions. By isolating each unit of code, unit tests help identify and fix potential bugs early in the development process, preventing them from propagating into larger and more complex components.
Integration tests, on the other hand, assess the interactions and dependencies between different modules or components. They ensure that the code functions seamlessly as a cohesive unit, identifying any issues that may arise when multiple components are integrated together. In the context of discount calculations, integration tests play a crucial role in verifying the correct flow of data between different functions and modules, ensuring that discounts are applied accurately and consistently.
Real-life examples abound, consider an e-commerce application where discounts are calculated based on various criteria, such as membership status, coupon codes, and product categories. Unit tests would be employed to validate the individual components responsible for calculating discounts for each criterion, ensuring their correctness and adherence to business rules. Integration tests would then be used to verify that these components work together seamlessly, ensuring that the overall discount calculation process is accurate and reliable.
Documentation
Documentation plays a pivotal role in the context of “how to calculate discount in python” by providing comprehensive guidance and real-world examples to users. It ensures that developers and users can effectively understand, implement, and utilize the code for accurate discount calculations.
- Tutorials: Step-by-step guides that demonstrate the practical application of discount calculation functions, complete with code snippets and explanations.
- Code Examples: Self-contained code samples that showcase specific scenarios and use cases, allowing users to adapt them to their own projects.
- API Reference: Detailed documentation of the available functions and methods for discount calculations, including their syntax, parameters, and return values.
- FAQs and Troubleshooting: Answers to frequently asked questions and guidance on resolving common issues encountered during discount calculations.
These facets of documentation are essential for enabling users to grasp the intricacies of discount calculations in Python. They provide a solid foundation for understanding the underlying concepts, implementing the code correctly, and troubleshooting any challenges that may arise. Comprehensive documentation not only enhances the usability of the code but also fosters a deeper understanding of the subject matter.
Frequently Asked Questions
This section addresses common questions and misconceptions surrounding discount calculations in Python, providing clear and concise answers to enhance comprehension.
Question 1: How do I calculate the discount amount?
To calculate the discount amount, multiply the original price by the discount rate. The discount rate should be expressed as a decimal (e.g., 10% discount would be 0.10).
Question 2: What is the difference between a discount and a sale price?
A discount is a reduction applied to the original price, while a sale price is the final price after the discount has been applied. Discounts are typically expressed as a percentage, while sale prices are absolute values.
Question 3: How do I apply multiple discounts?
When applying multiple discounts, the order of application matters. Discounts can be chained or applied simultaneously, depending on the specific requirements. Careful consideration should be given to the order of operations to achieve the desired result.
Question 4: Can I calculate discounts for bulk purchases?
Yes, it is possible to calculate discounts for bulk purchases by applying a tiered discount structure. This involves setting different discount rates based on the quantity of items purchased.
Question 5: How do I handle negative values for price or discount rate?
Negative values for price or discount rate should be handled appropriately to avoid errors. Robust code should include checks to ensure that these values are positive or zero.
Question 6: What are some common pitfalls to avoid when calculating discounts?
Common pitfalls include using incorrect data types, neglecting to handle edge cases, and applying discounts incorrectly. Careful attention to detail and thorough testing can help avoid these issues.
These FAQs provide valuable insights into the nuances of discount calculations in Python. Understanding these concepts will help developers implement accurate and reliable discount calculations in their applications.
The next section of this article will delve into advanced topics related to discount calculations, including strategies for optimizing performance and handling complex scenarios.
Tips for Calculating Discounts in Python
To enhance proficiency in calculating discounts in Python, consider these practical tips:
Tip 1: Utilize Built-in Functions: Leverage Python’s math library for mathematical operations, including rounding and power calculations.
Tip 2: Choose Appropriate Data Types: Select data types (e.g., float or decimal) based on the precision and range required for discount calculations.
Tip 3: Employ Clear Variable Names: Use descriptive variable names to enhance code readability and maintainability.
Tip 4: Implement Error Handling: Handle invalid inputs and negative values to prevent errors and ensure robust code.
Tip 5: Optimize Code for Performance: Employ efficient algorithms and data structures to minimize execution time, particularly for large datasets.
Tip 6: Write Comprehensive Tests: Conduct unit and integration tests to verify the accuracy and reliability of discount calculations.
Tip 7: Provide Clear Documentation: Document code with tutorials, examples, and API references to facilitate understanding and usage.
By applying these tips, developers can refine their Python code for discount calculations, achieving greater precision, efficiency, and maintainability.
In the concluding section, we will explore advanced strategies for handling complex discount scenarios and optimizing performance for large-scale applications.
Conclusion
This article has delved into the intricacies of “how to calculate discount in Python,” providing a comprehensive guide for developers to implement accurate and reliable discount calculations in their applications. Key takeaways include:
- Understanding the core formula (discount = price * discount_rate) and utilizing Python’s built-in math library for mathematical operations.
- Selecting appropriate data types and employing clear variable names to enhance code readability and maintainability.
- Implementing robust error handling mechanisms to prevent unexpected behavior and ensure the integrity of discount calculations.
As businesses increasingly rely on data-driven insights and automated processes, mastering discount calculations in Python becomes a valuable skill for data analysts, financial professionals, and software engineers alike. By leveraging the techniques outlined in this article, developers can confidently tackle complex discount scenarios, optimize their code for performance, and deliver reliable results in their applications.
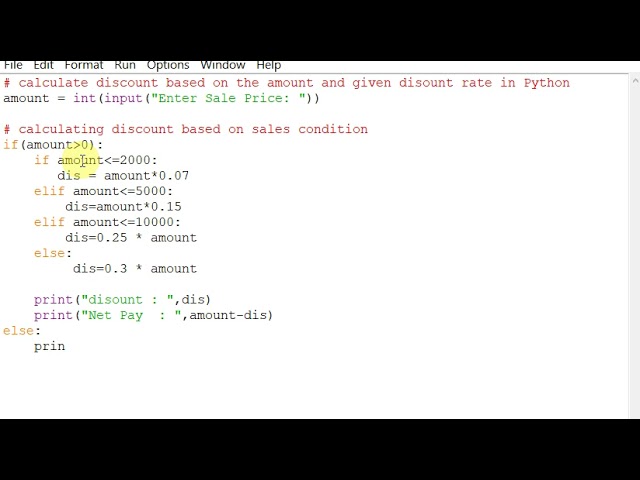